Matplotlibの極座標系の図#
※記事内に商品プロモーションを含むことがあります。
公開日
極座標系の図をプロットするには、plt.subplots()
のsubplot_kw
オプションに{'projection': 'polar'}
という辞書形式で与えます。
このとき、ax.plot()
の最初の引数に角度[rad], 2番目の引数に原点からの距離を与えます。
import numpy as np
import matplotlib.pyplot as plt
theta = np.pi*np.arange(0, 3, 0.01)
r = np.arange(0, 3, 0.01)
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
ax.plot(theta, r)
plt.show()
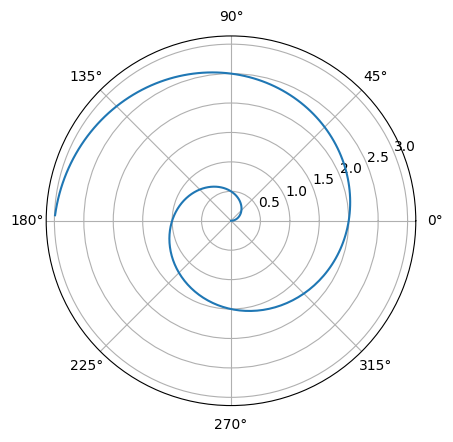
軸の範囲#
角度方向の軸の範囲はax.set_thetalim()
, 動径方向の軸の範囲はax.set_rlim()
でそれぞれ設定します。
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
ax.plot(theta, r)
ax.set_thetalim(0, np.pi)
ax.set_rlim(-3, 3)
plt.show()
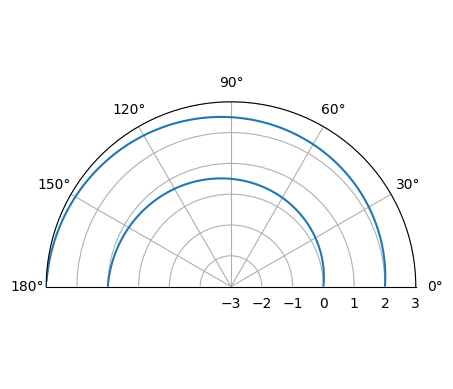
軸の目盛り#
偏角方向#
偏角方向の軸の目盛りを設定するには、ax.set_thetagrids()
を使用します。表示したい目盛りの角度[deg]を配列で与えます。
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
ax.plot(theta, r)
ax.set_thetagrids([0, 90, 180, 270])
plt.show()
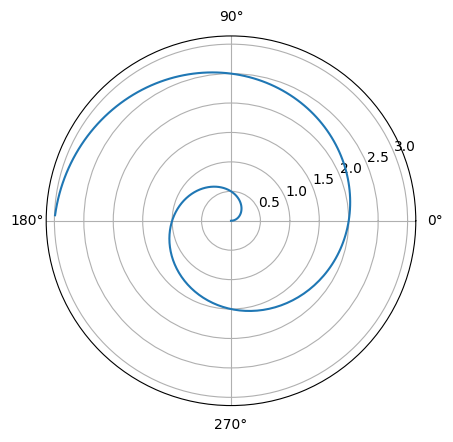
labels
オプションで任意の目盛りラベルを設定できます。
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
ax.plot(theta, r)
ax.set_thetagrids([0, 90, 180, 270], labels=["E", "N", "W", "S"])
plt.show()
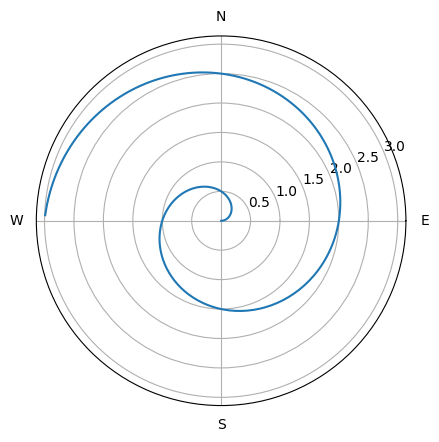
動径方向#
動径方向の軸の目盛りを設定するには、ax.set_rgrids()
を使用します。表示したい目盛りの位置を配列で与えます。
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
ax.plot(theta, r)
ax.set_rgrids([0, 1, 2, 3])
plt.show()
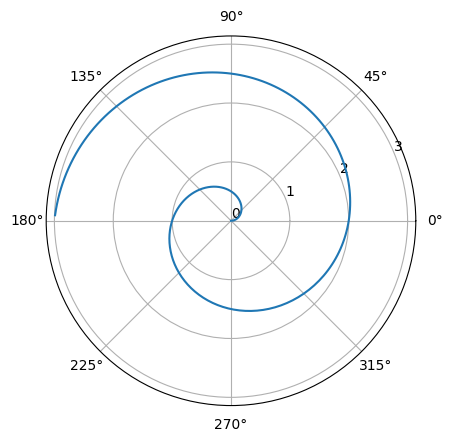
さらに、labels
オプションで任意の目盛りラベルを、angle
で目盛りを表示する角度[deg]をそれぞれ設定できます。
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
ax.plot(theta, r)
ax.set_rgrids([0, 1, 2, 3], labels=["a", "b", "c", "d"], angle=60)
plt.show()
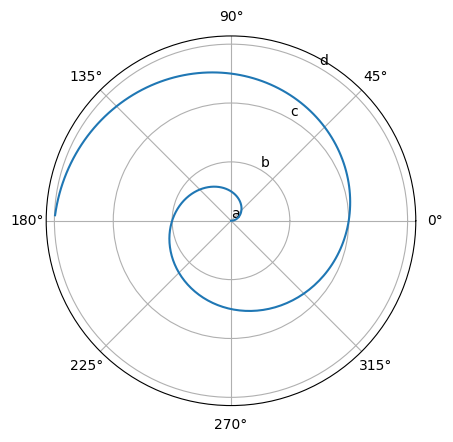
偏角方向の軸の始点#
ax.set_theta_zero_location()
によって、角度方向の軸の始点を指定できます。引数は方角であり、"N", "NW", "W", "SW", "S", "SE", "E", "NE"のいずれかを与えます。さらにoffset
オプションで半時計回りの回転角度[deg]を指定します(角度方向の軸の向きによらず、常に半時計回りの回転角度になります)。
以下は"N"で真上を始点とした例です。
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
ax.plot(theta, r)
ax.set_theta_zero_location("N")
plt.show()
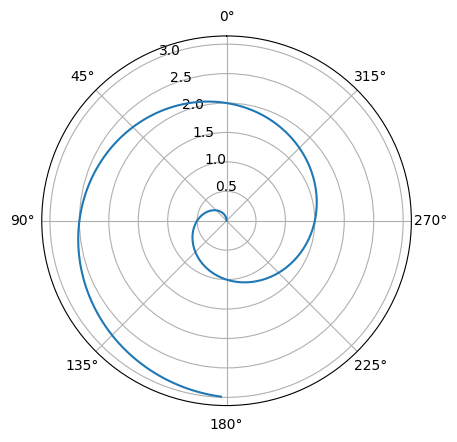
始点を真上からさらに半時計回りに10度回転させた例を以下に示します。
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
ax.plot(theta, r)
ax.set_theta_zero_location("N", offset=10)
plt.show()
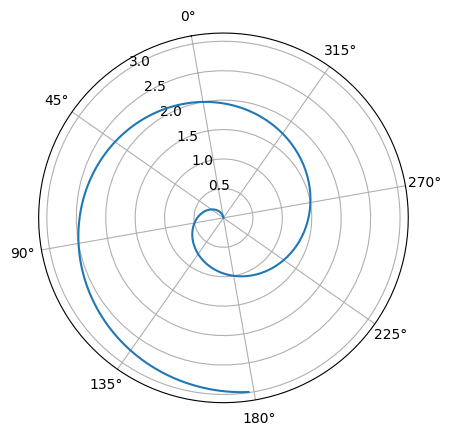
動径方向の軸の始点#
ax.set_rorigin()
に負の値を指定することで、動径方向の原点を外側にずらせます。つまり、ドーナツグラフのような見た目になります。
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
ax.plot(theta, r)
ax.set_rorigin(-1)
plt.show()
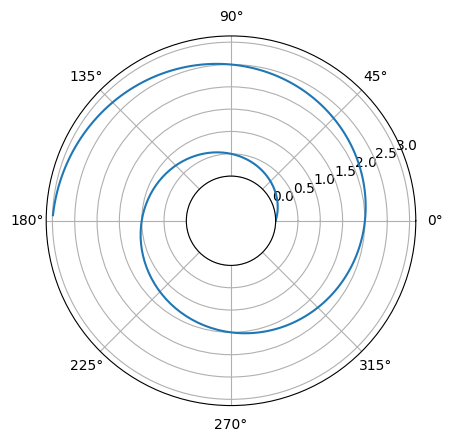
偏角の回転方向#
偏角の回転方向を反対(時計回り)にするには、ax.set_theta_direction(-1)
を与えます。引数を1
にすると、通常通り半時計回りとなります。
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
ax.plot(theta, r)
ax.set_theta_direction(-1)
plt.show()
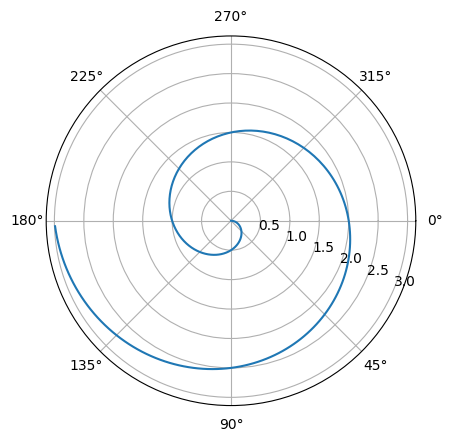
極座標系の様々なプロット#
棒グラフ#
極座標系の棒グラフをプロットするには、ax.bar()
を用います。width
オプションで棒の幅、alpha
オプションで棒の色の透明度を指定できます。
theta2 = np.pi*np.arange(0, 2, 0.5)
r2 = np.arange(1, 3, 0.5)
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
ax.bar(theta2, r2, width=0.3, alpha=0.7)
ax.bar(theta2+0.2, r2+0.5, width=0.3, alpha=0.7)
plt.show()
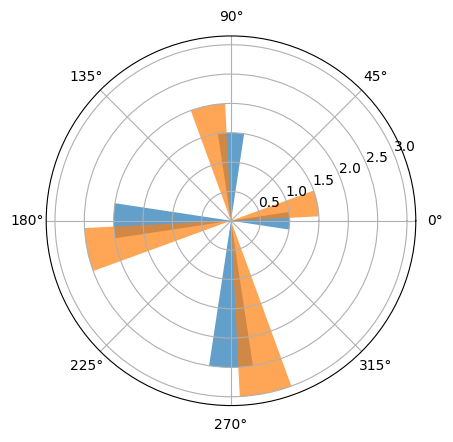
散布図#
極座標系の散布図をプロットするには、ax.scatter()
を用います。マーカーの大きさはs
オプションで指定できます。その他のオプションについては散布図を参照して下さい。
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
ax.scatter(theta2, r2)
ax.scatter(theta2+0.2, r2+0.5, s=100)
plt.show()
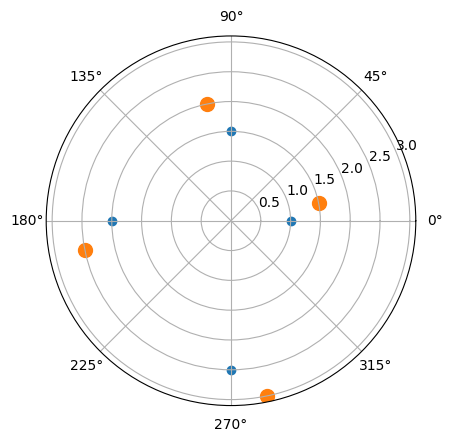
ヒートマップ#
極座標系のヒートマップをプロットするには、ax.pcolor()
を用います。最初の引数は角度[rad], 2番目の引数は動径、3番目の引数は色に対応するデータ(動径データ数×角度データ数の2次元配列)とします。
theta3 = np.linspace(0, 2*np.pi, 30) # データ数30の1次元配列(角度)
r3 = np.linspace(0, 100, 10) # データ数10の1次元配列(動径)
C = np.random.random_sample((10, 30)) # 10x30の2次元配列
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
mappable = ax.pcolor(theta3, r3, C, shading="auto")
plt.colorbar(mappable, ax=ax)
plt.show()
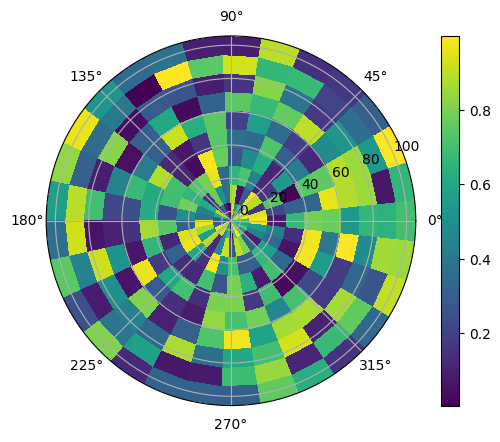
ax.pcolor()
のcmap
オプションでカラーマップを指定できます。指定可能なカラーマップについては、以下のページも参照下さい。
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
mappable = ax.pcolor(theta3, r3, C, shading="auto", cmap="Blues")
plt.colorbar(mappable, ax=ax)
plt.show()
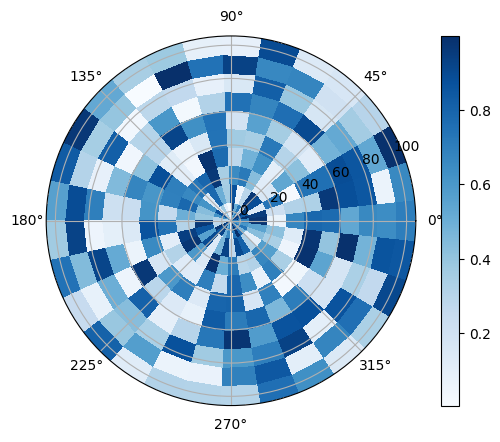