Matplotlibの目盛りの設定#
※記事内に商品プロモーションを含むことがあります。
公開日
Matplotlibの目盛りの設定(補助目盛りや目盛り線など)について解説します。
補助目盛り#
補助目盛りを表示するには、Axes.minorticks_on()
メソッドを用います。
import matplotlib.pyplot as plt
x = [1, 3, 2]
# 補助目盛りなし
fig, ax = plt.subplots()
ax.plot(x)
plt.show()
# 補助目盛りあり
fig, ax = plt.subplots()
ax.plot(x)
ax.minorticks_on()
plt.show()
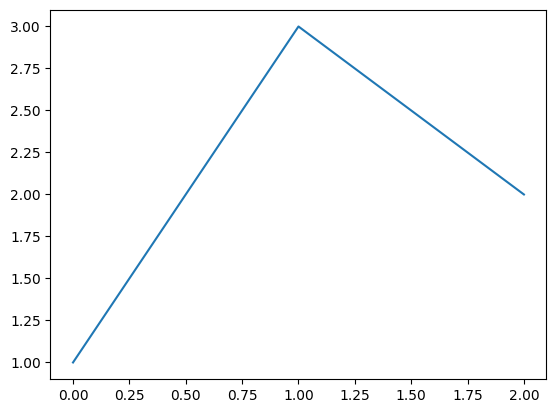
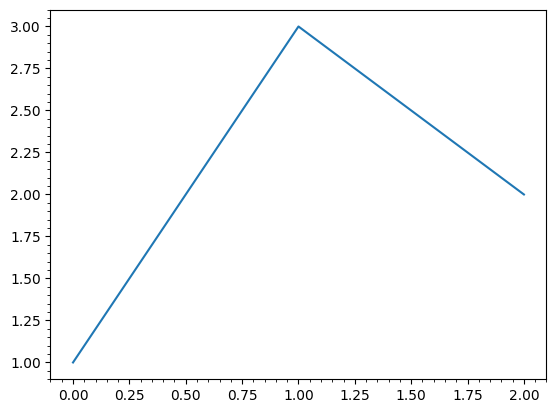
目盛り線#
目盛り線を引くには、Axes.grid()
メソッドを用います。このメソッドの主なオプションは以下の通りです。
オプション |
型 |
説明 |
---|---|---|
axis |
str |
軸を選択('both', 'x', 'y') |
which |
str |
目盛り線の種類を指定。'major'(主目盛り)、'minor'(補助目盛り)、'both'(両方の目盛り) |
linewidth |
float |
線の太さ |
color |
str |
線の色 |
linestyle |
str |
線の種類 |
axis
オプションを使ってx軸のみ目盛り線を引く例を以下に示します。
fig, ax = plt.subplots()
ax.plot([1, 3, 2])
ax.grid()
plt.show()
# x軸のみ目盛り線を引く
fig, ax = plt.subplots()
ax.plot([1, 3, 2])
ax.grid(axis="x")
plt.show()
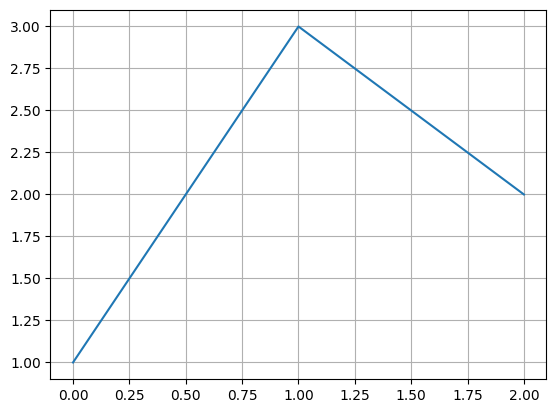
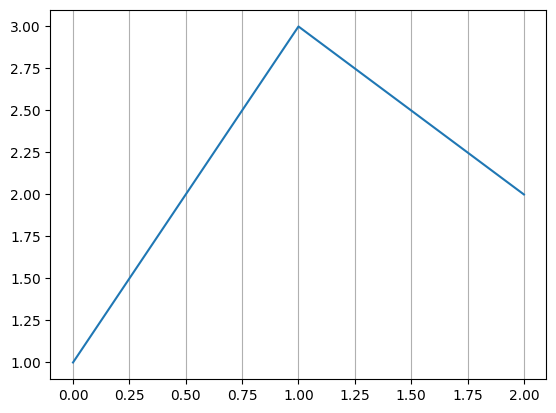
grid()
メソッドのwhich
オプションを指定しない場合、主目盛り線のみ引かれます。補助目盛りにも線を引くには、ax.minorticks_on()
で補助目盛りをONにした後、ax.grid(which="both")
を実行します。
fig, ax = plt.subplots()
ax.plot([1, 3, 2])
ax.minorticks_on() # 補助目盛りを表示する
ax.grid() # デフォルトでは主目盛り線のみ表示される
plt.show()
# 主目盛りと補助目盛りの両方に目盛り線を引く
fig, ax = plt.subplots()
ax.plot([1, 3, 2])
ax.minorticks_on()
ax.grid(which="both")
plt.show()
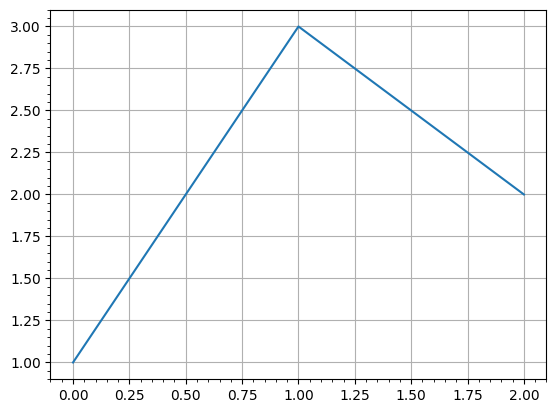
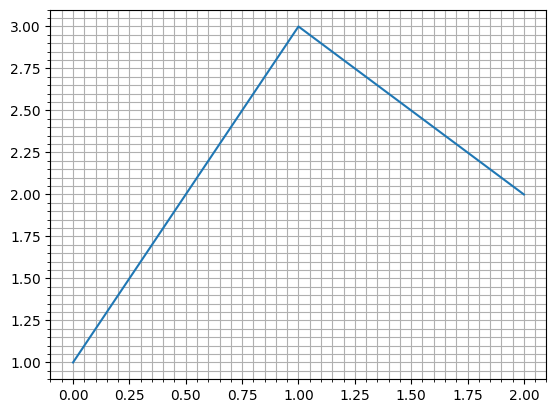
目盛り線の太さはlinewidth
オプション、色はcolor
オプション、種類はlinestyle
オプションでそれぞれ変更できます。例を以下に示します。
fig, ax = plt.subplots()
ax.plot([1, 3, 2])
ax.grid(linewidth=1.5, color="green", linestyle="--")
plt.show()
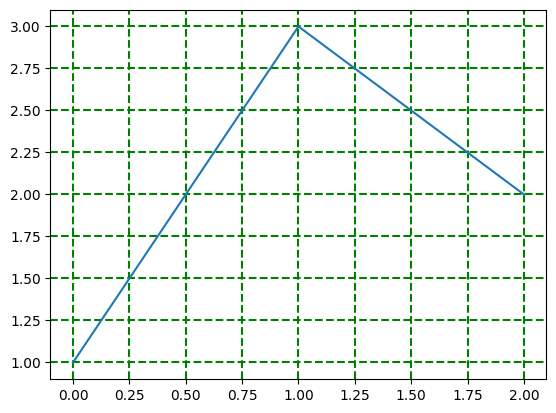
tick_params#
目盛りの詳細な設定をするには、Axes.tick_params()
メソッドを用います。このメソッドの主なオプションを以下に示します。
オプション |
型 |
説明 |
---|---|---|
axis |
str |
軸を選択('both', 'x', 'y') |
which |
str |
目盛り線の種類を指定。'major'(主目盛り)、'minor'(補助目盛り)、'both'(両方の目盛り) |
direction |
str |
目盛りの位置。'in', 'out', 'inout' |
length |
float |
目盛りの長さ |
width |
float |
目盛りの幅 |
color |
str |
目盛りの色 |
pad |
float |
目盛りと目盛りラベルの隙間 |
labelsize |
float |
目盛りラベルの大きさ |
labelcolor |
str |
目盛りラベルの色 |
colors |
str |
目盛りと目盛りラベルの色 |
zorder |
float |
描画する順序 |
labelrotation |
float |
目盛りラベルの回転角度(半時計回りに回転。単位は度) |
なお、Axes.tick_params()
メソッドで補助目盛りの設定をする場合、Axes.minorticks_on()
メソッドを実行する必要があります。
目盛りの向き#
目盛りの向きはdirection
オプションで指定できます。in
で内側、out
で外側(デフォルト)、inout
で両方となります。
# 内側
fig, ax = plt.subplots()
ax.plot([1, 3, 2])
ax.tick_params(direction='in')
plt.show()
# 両方
fig, ax = plt.subplots()
ax.plot([1, 3, 2])
ax.tick_params(direction='inout')
plt.show()
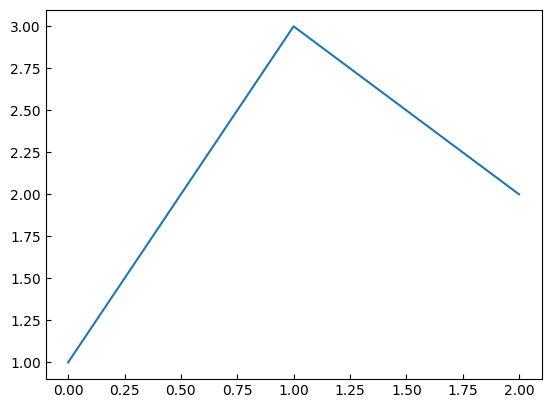
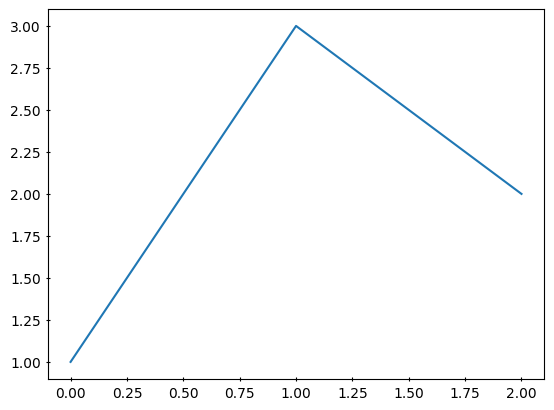
目盛りとラベルの色#
color
オプションでは目盛り線のみ、colors
オプションでは目盛り線と目盛りラベルの両方の色を変更できます。また、labelcolor
オプションでは、目盛りラベルのみ色を変更できます。
# 目盛り線を赤色に設定
fig, ax = plt.subplots()
ax.plot([1, 3, 2])
ax.tick_params(color="red", width=3)
plt.show()
# 目盛り線と目盛りラベルを赤色に設定
fig, ax = plt.subplots()
ax.plot([1, 3, 2])
ax.tick_params(colors="red", width=3)
plt.show()
# 目盛りラベルを赤色に設定
fig, ax = plt.subplots()
ax.plot([1, 3, 2])
ax.tick_params(labelcolor="red", width=3)
plt.show()
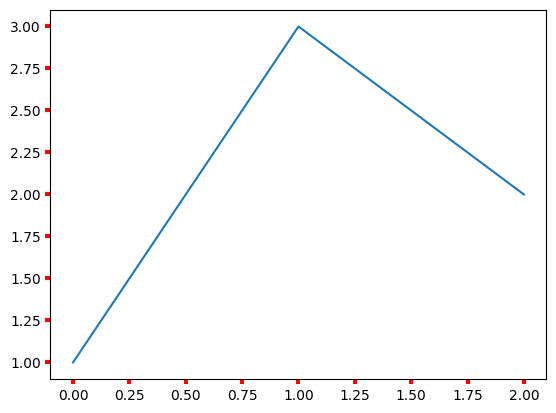
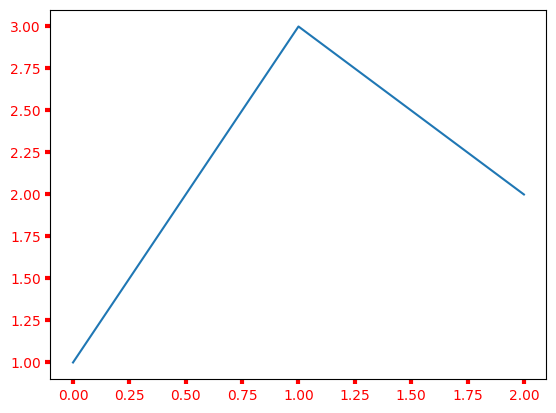
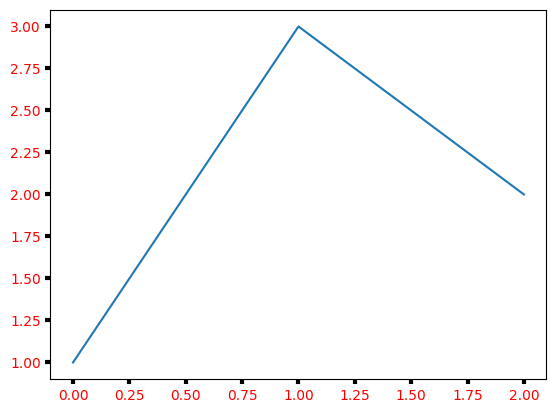
目盛りラベルの回転#
labelrotation
オプションによって目盛りラベルを回転できます。半時計回りに回転し、単位は度です。
fig, ax = plt.subplots()
ax.plot([1, 3, 2])
ax.tick_params(labelrotation=45)
plt.show()
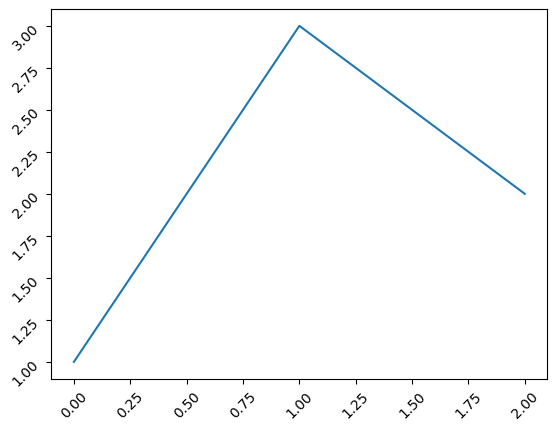
x軸またはy軸のみの目盛りラベルを回転させる場合、ax.tick_params()
メソッドのaxis
オプションで指定します。
fig, ax = plt.subplots()
ax.plot([1, 3, 2])
ax.tick_params(axis="x", labelrotation=45)
ax.tick_params(axis="y", labelrotation=-10)
plt.show()
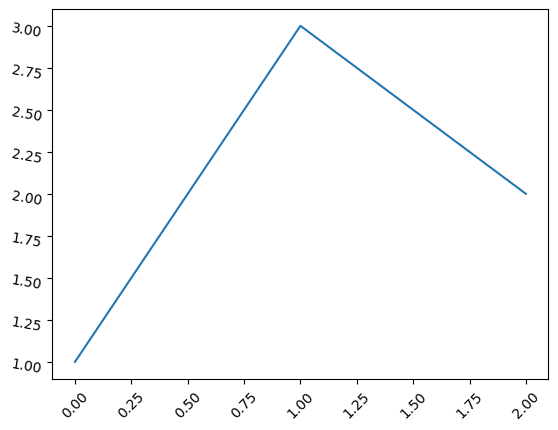